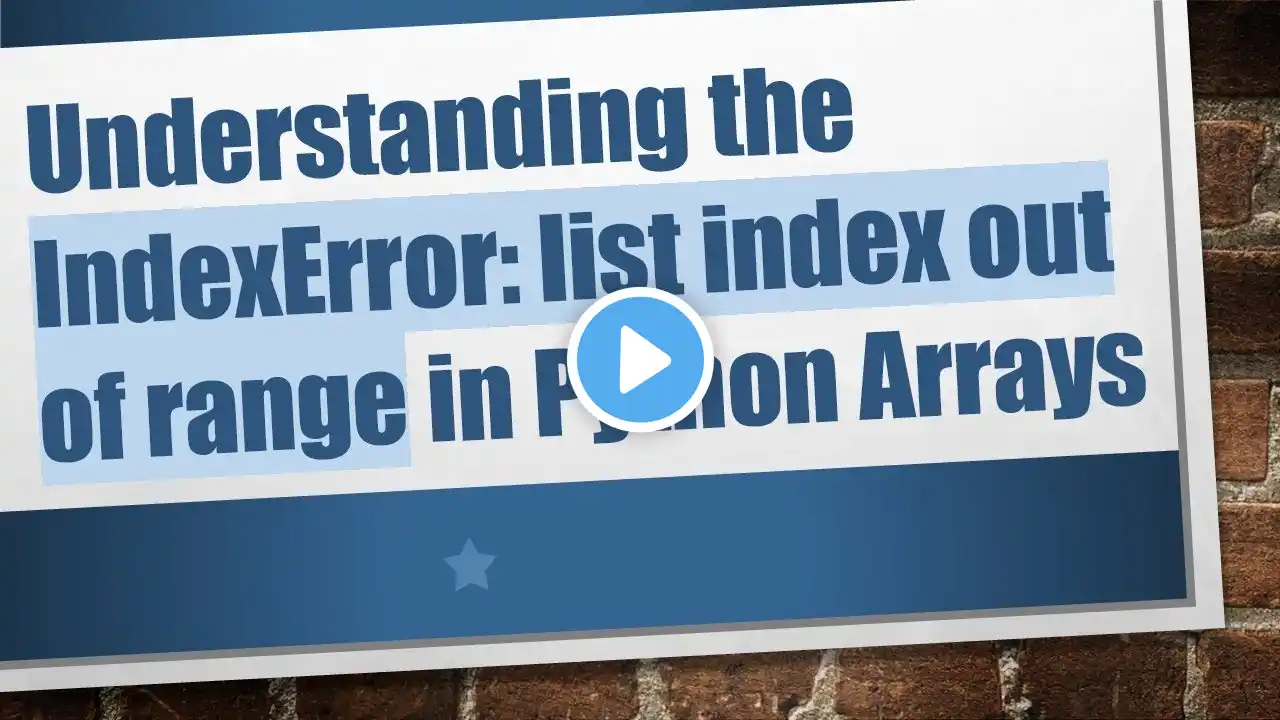
Understanding the IndexError: list index out of range in Python Arrays
Learn how to fix the common `IndexError` that occurs in Python when working with arrays and loops. This guide explains the issue and provides effective solutions. --- This video is based on the question https://stackoverflow.com/q/68873022/ asked by the user 'Omar Ayman Ayoub' ( https://stackoverflow.com/u/14579723/ ) and on the answer https://stackoverflow.com/a/68873056/ provided by the user 'quamrana' ( https://stackoverflow.com/u/4834/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Why this happens when there is a condition prevents it Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- Understanding the IndexError in Python Arrays Programming in Python can be quite rewarding, but it can also come with its own set of challenges. One common error encountered by beginners is the dreaded IndexError: list index out of range. This error often occurs when working with arrays (or lists in Python), especially when using loops and conditions without proper checks. In this guide, we will explore why this error happens and how to effectively resolve it. The Problem: What Causes the IndexError? Let’s consider the following code snippet that leads to the IndexError: [[See Video to Reveal this Text or Code Snippet]] Breakdown of the Issue Input Handling: The code starts by prompting the user for the number of inputs and collects them into a list called Numbers. Looping with Conditions: It attempts to iterate through the list using nested conditions. The critical problem arises during the comparison check if(Numbers[i] >= Numbers[i + 1]), particularly for the last element in the list. The Python Error IndexError: list index out of range occurs specifically because Numbers[i + 1] tries to access an index that does not exist. If i equals the last index of the list, i + 1 exceeds the length of the list, leading to this error. The Solution: Preventing Index Errors To avoid running into this issue, you can modify your loop conditions. Here are two effective solutions: Solution 1: Adjust the Loop Condition Change the loop limit to x - 1 to ensure you don't access an out-of-bounds index: [[See Video to Reveal this Text or Code Snippet]] Solution 2: Include a Break Condition Alternatively, if you prefer to keep your loop at x iterations, ensure you check against the last index: [[See Video to Reveal this Text or Code Snippet]] Why These Changes Work Both solutions effectively prevent accessing an index that is out of the list’s range. Using x - 1 in the loop ensures that every valid index can safely access Numbers[i + 1]. The break statement checks if you are at the last index and prevent further execution of the condition check. Conclusion The IndexError: list index out of range can certainly be frustrating, but understanding the reason for its occurrence allows you to implement simple fixes. By correctly managing loop conditions and ensuring you stay within the bounds of your lists, you can avoid these errors and write more robust code. Remember to always test your loops when handling lists to catch potential index issues early on in your coding journey. Happy coding!