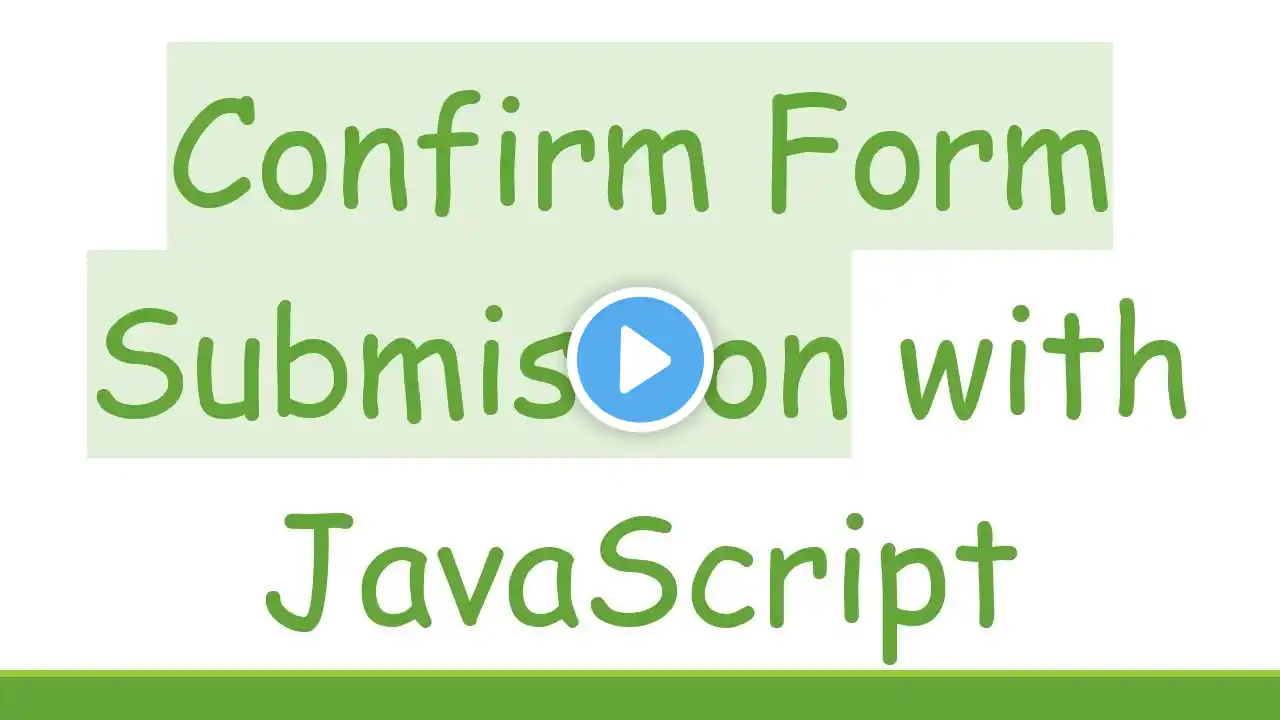
Confirm Form Submission with JavaScript
Learn how to implement a JavaScript confirmation message to halt form submissions effectively. Enhance user experience by ensuring intentional action with simple code! --- This video is based on the question https://stackoverflow.com/q/76337800/ asked by the user 'kbdev' ( https://stackoverflow.com/u/3890615/ ) and on the answer https://stackoverflow.com/a/76337811/ provided by the user 'Anish Chittora' ( https://stackoverflow.com/u/13711324/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Javascript comfirmation message to stop form submission Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- Confirm Form Submission with JavaScript: A Complete Guide When designing web forms, it's vital to ensure that users are consciously submitting their data. You may have encountered a scenario where someone accidentally submits a form, leading to frustration and wasted time. To prevent this, you can implement a JavaScript confirmation message that prompts the user to verify their decision before the form is submitted. This guide will guide you through creating such a confirmation mechanism step-by-step. The Problem You want users to have the option to confirm their intention to submit a form. When they click the "submit" button, a message should pop up asking if they are sure they want to proceed. If the user clicks "Cancel," the submission should be halted. The Initial Attempt and its Flaw Many developers starting out may try to implement this feature with the following code: [[See Video to Reveal this Text or Code Snippet]] Although this code seems sound, it contains a critical error: the event listener is incorrectly listening for onsubmit instead of submit. This mistake leads to the functionality not working at all. Let's correct that by focusing on the right approach. The Correct Approach Step 1: Select the Form Element Make sure you target the correct form element on your webpage. Your JavaScript code should begin with selecting the form using document.querySelector, ensuring that the form ID matches what is present in your HTML. Step 2: Implement the Event Listener Next, you'll want to attach the submit event listener correctly. Here is how you can do it: [[See Video to Reveal this Text or Code Snippet]] Breakdown of the Correct Code Selecting the Form: The line const form = document.querySelector('-incidentform'); selects the form with the ID incidentform. Event Listener: The line form.addEventListener('submit', function(event) {...}); attaches an event listener for the submit event. Confirmation Box: The confirm(text) function displays a modal dialog with your message, allowing users to either cancel or confirm the submission. Prevent Default Submission: The line event.preventDefault(); is vital. If the user cancels the confirmation dialog, this function stops the form from being submitted. Final Thoughts By following this guide, you'll ensure that users are prompted to confirm their submission decision, leading to a better user experience and fewer accidental submissions. Always test your forms after implementing changes to ensure everything works as expected. Feel free to adapt and expand upon the provided code to suit your website's design and functionality needs. Enabling confirmation messages is a small yet effective step toward improving your web forms!