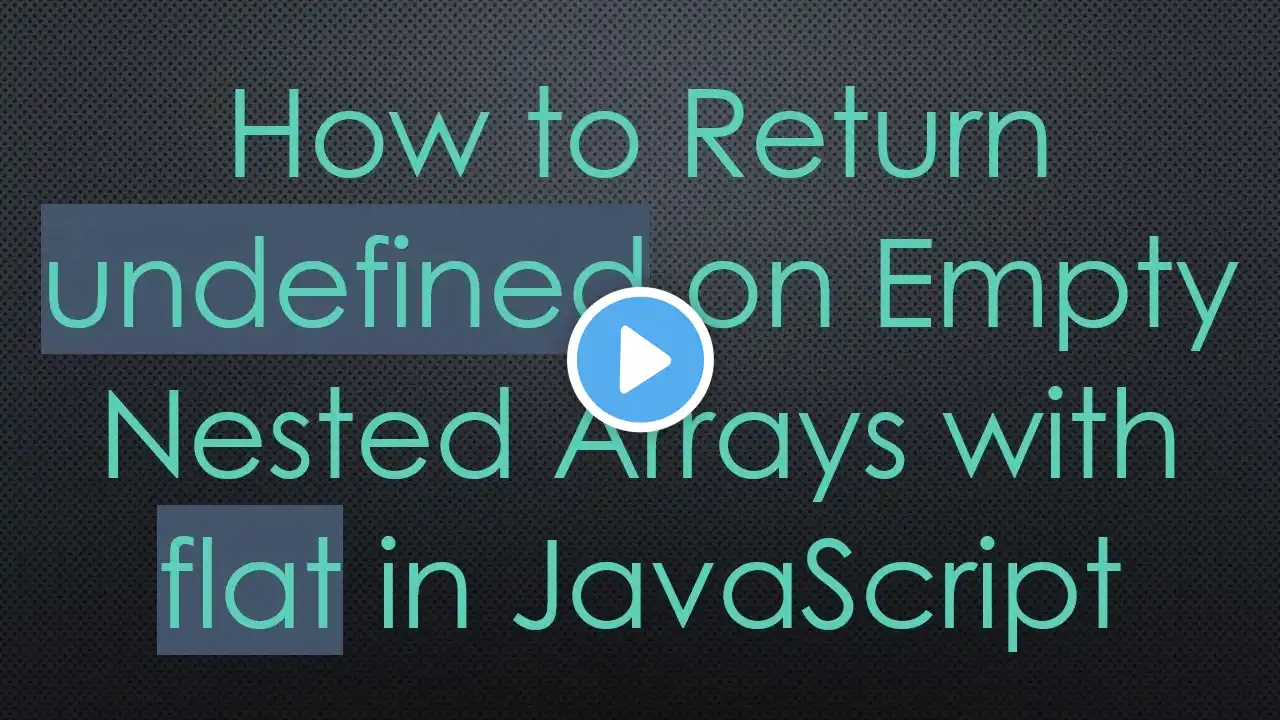
How to Return undefined on Empty Nested Arrays with flat in JavaScript
Learn how to effectively handle empty nested arrays in JavaScript by using the `flatMap` method to return `undefined` values instead of an empty array. --- This video is based on the question https://stackoverflow.com/q/73813002/ asked by the user 'sir-haver' ( https://stackoverflow.com/u/9856049/ ) and on the answer https://stackoverflow.com/a/73813044/ provided by the user 'Anton Marinenko' ( https://stackoverflow.com/u/11248646/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Return undefined on empty nested arrays with flat Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- Handling Empty Nested Arrays in JavaScript When working with arrays in JavaScript, especially nested arrays, it can often get complicated. A common scenario occurs when you want to count the number of items, including undefined values, within nested arrays. For instance, if you have a nested array that is entirely empty, how can you ensure that it returns undefined values instead of just yielding an empty array? In this guide, we'll explore a straightforward solution using the flatMap method. The Problem Consider the following situations with nested arrays: When using the flat() method on the array [[undefined, 4], [4]], you receive three items: undefined 4 4 However, if you attempt to flatten the array [[], [], []], which consists purely of empty arrays, the flat() method returns an empty array, not the three undefined values you might expect. This can be frustrating if you're aiming for consistency in your data handling and would like to preserve the count of supposed empty slots. The Solution To achieve the desired outcome where empty arrays return undefined, you can use the flatMap() method, which gives you more control over the transformation of your array elements before flattening them. Here’s how you can implement it. Step-by-Step Implementation Understanding flatMap(): This method maps each element using a mapping function, and then flattens the result into a new array. This allows you to define exactly what happens to each item. Writing Your Function: Utilize a mapping function to check if each array is empty: [[See Video to Reveal this Text or Code Snippet]] Explanation: item.length ? item : undefined: This checks if the current item has any length. If it does (i.e., it's not empty), it returns the item; otherwise, it returns undefined. The result of this flatMap call will ensure that each empty array corresponds to an undefined value. Example Let’s look at this in a practical context. Here’s how you would implement this in code: [[See Video to Reveal this Text or Code Snippet]] This approach keeps the output consistent even when facing empty arrays, preserving the structural integrity of the expected results. Conclusion Using flatMap() gives you a straightforward solution to the issue of handling empty nested arrays in JavaScript. By leveraging this method, you can ensure that an empty nested array returns undefined values, allowing you to maintain a more predictable behavior in your code. Next time you encounter empty nested arrays, remember this approach to keep your data handling consistent and clear! With this understanding, you are now equipped to tackle similar challenges effectively and make your JavaScript code cleaner and more reliable.