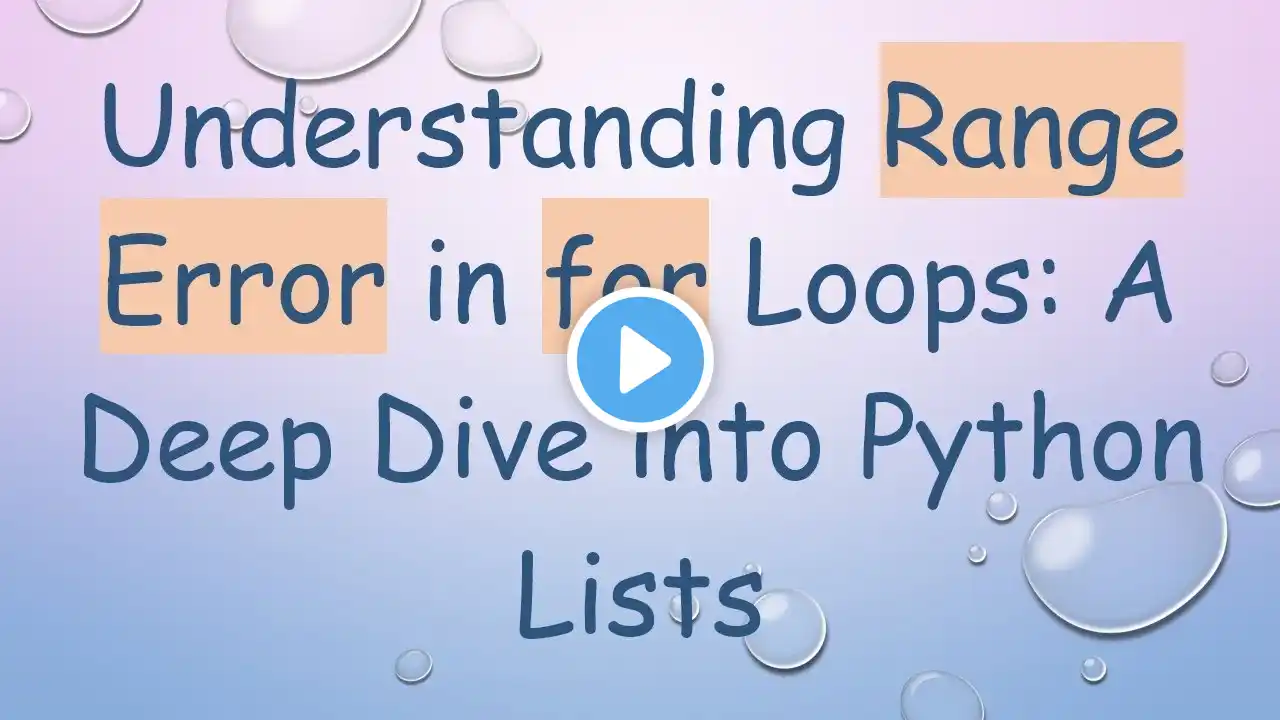
Understanding Range Error in for Loops: A Deep Dive into Python Lists
Discover why range errors occur in Python for loops with this comprehensive guide. Learn how to manage nested lists, fix your code, and prevent out of range errors. --- This video is based on the question https://stackoverflow.com/q/78065728/ asked by the user 'Jsinotte' ( https://stackoverflow.com/u/23472156/ ) and on the answer https://stackoverflow.com/a/78065849/ provided by the user 'balintd' ( https://stackoverflow.com/u/13134286/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Why is there a range error in the for loop? Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- Understanding Range Error in for Loops: A Deep Dive into Python Lists When programming in Python, encountering a range error can be frustrating, particularly in the context of a for loop. This guide addresses a common scenario that leads to this error and guides you through the steps to resolve it effectively. Let’s dive into the specifics! The Problem You might be working on an assignment where you need to create a function that analyzes the frequency of characters in a string without using built-in functions other than len(). In your code, you're attempting to populate a list with individual characters from the provided string. However, an unexpected range error occurs, particularly after the first iteration of your loop. The following code snippet illustrates the issue: [[See Video to Reveal this Text or Code Snippet]] Input: "fhh" [[See Video to Reveal this Text or Code Snippet]] The Root Cause The error arises because you're inadvertently creating a nested list when defining freq_list. The structure of freq_list is as follows: [[See Video to Reveal this Text or Code Snippet]] Breaking Down the Issue: Nested List: The first element (freq_list[0]) is a list containing another list. This means when you try to access elements with freq_list[0][i], you're attempting to access the first index of a nested list, which doesn't contain the individual positions you expect. Index Out of Range: As the for loop iterates through the indices, on your second iteration (i=1), the program tries to access freq_list[0][1], which is not actually available, resulting in an index out of range error. The Solution To fix the range error, you have two primary options: Option 1: Correcting the List Definition If the nested list is not required, you can redefine your freq_list like this: [[See Video to Reveal this Text or Code Snippet]] Option 2: Accessing the Inner List If you intend to keep the nested structure, you should adjust your assignments to: [[See Video to Reveal this Text or Code Snippet]] This approach correctly accesses the inner list, populating it with the characters from the input string. Conclusion Understanding how list structures work in Python is crucial to avoiding errors, especially range errors in loops. By correcting the way we manage our lists—whether flattening a nested structure or accurately indexing into it—programmers can prevent these common pitfalls. Key Takeaways Always be aware of the structure you use when creating lists. Adjust your indexing based on whether you intended to create a nested list. Test your code with different inputs to catch any potential errors. By implementing these suggestions, you can enhance your programming skills and reduce the likelihood of encountering similar issues in the future. Happy coding!