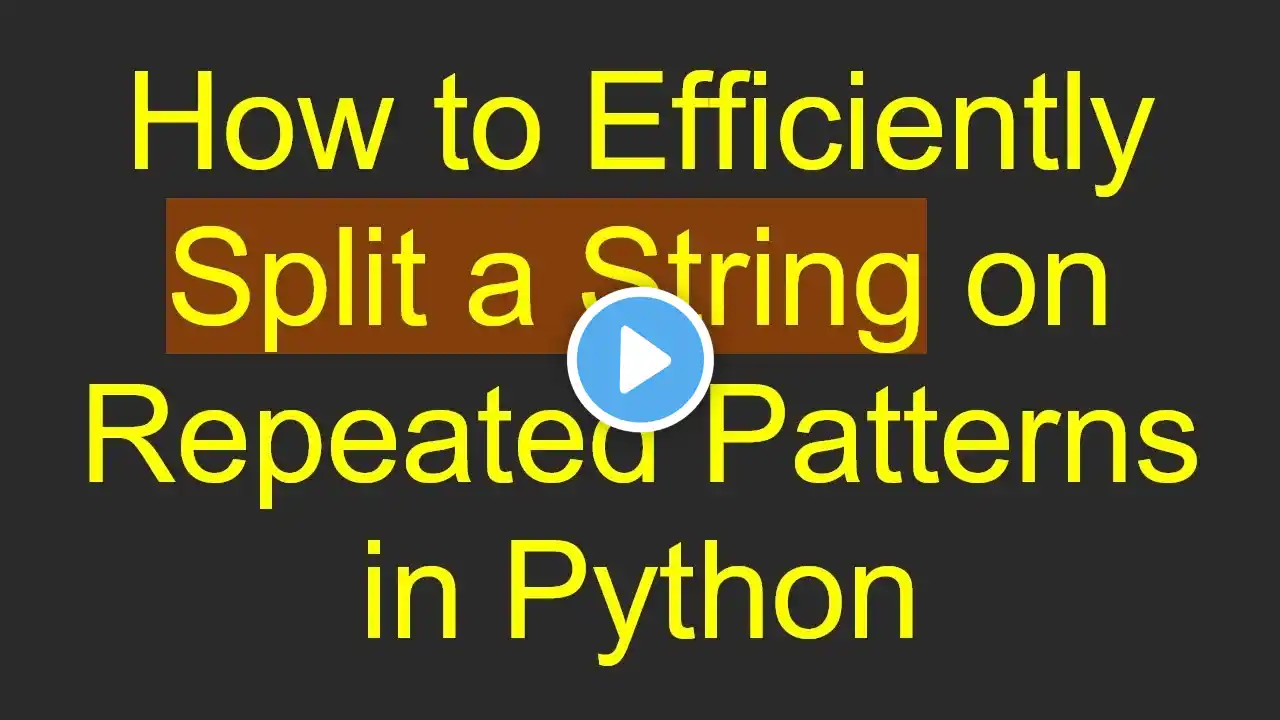
How to Efficiently Split a String on Repeated Patterns in Python
Learn how to split and remove repeated patterns from a string in Python. This guide will help you manage string data effectively using simple methods. --- This video is based on the question https://stackoverflow.com/q/75700411/ asked by the user 'John Stud' ( https://stackoverflow.com/u/6534818/ ) and on the answer https://stackoverflow.com/a/75700710/ provided by the user 'Swifty' ( https://stackoverflow.com/u/20267366/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python: str split on repeated instances in single string Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- How to Efficiently Split a String on Repeated Patterns in Python Handling strings in Python can sometimes be challenging, especially when dealing with repeated patterns. In this guide, we’ll address a common problem: how to split and remove repeated patterns from a complex string. This can be useful in various situations, like cleaning up log files or organizing command strings. The Problem Imagine you have a complex string that contains repeated patterns you wish to eliminate. Here’s a sample string: [[See Video to Reveal this Text or Code Snippet]] Your goal is to transform the above string to something like this: [[See Video to Reveal this Text or Code Snippet]] The problem with many initial attempts is that they often lead to only capturing the last repeated value instead of all instances. Let's explore the solution to this problem in depth. The Solution Step 1: Splitting the String The first step is to split the string in a way that you can isolate the repeated patterns. You can do this by splitting the string around spaces, so all relevant parts become easy to handle. You can achieve this with the following code: [[See Video to Reveal this Text or Code Snippet]] How It Works Split the String: The first part of the command s1.split() divides the entire string into individual components based on spaces. Accessing the Relevant Segment: For each part resulting from the first split, we use .split('/.cache/')[-1] to access only the segment after the repeated pattern. Joining the Components: Finally, join() is used to combine all the processed parts back into a single string, with spaces restoring the originally intended format. Output Running the above code will give you the desired output as follows: [[See Video to Reveal this Text or Code Snippet]] Summary In just a few simple steps, you can split a complex string in Python and effectively remove unwanted repeated patterns. This method not only helps in cleaning up the data but also makes it easier to work with strings in your applications. By isolating parts of the string and then rejoining them, you can achieve neat and manageable output. Using the provided technique, you can handle your string manipulations in Python more confidently and effectively! Make sure to experiment with different strings and patterns to fully understand the flexibility of string manipulation capabilities in Python!