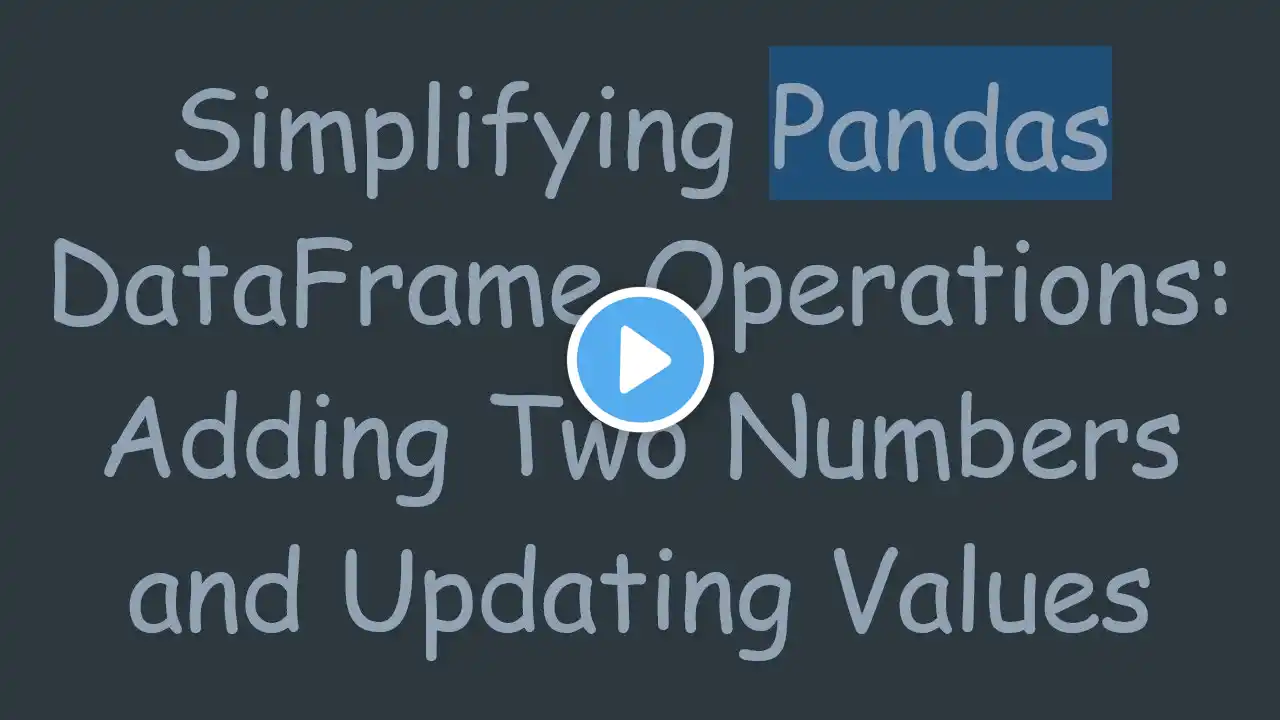
Simplifying Pandas DataFrame Operations: Adding Two Numbers and Updating Values
Learn how to easily add two numbers within a `Pandas` DataFrame and update the results with a simple one-liner using `lambda` functions. --- This video is based on the question https://stackoverflow.com/q/73502884/ asked by the user 'Andrew' ( https://stackoverflow.com/u/12280485/ ) and on the answer https://stackoverflow.com/a/73510524/ provided by the user 'Andrew' ( https://stackoverflow.com/u/12280485/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Pandas DataFrame adding of two numbers and updating it Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- Simplifying Pandas DataFrame Operations: Adding Two Numbers and Updating Values Working with data can sometimes lead to complex tasks that may initially feel overwhelming. For those using Pandas, a powerful data manipulation library in Python, there are often more straightforward solutions that can save you time and effort. One common task is to add two numbers together stored in a DataFrame and then update the results accordingly. In this guide, we’ll walk through a specific example to show you how to seamlessly perform this operation using Pandas and numpy libraries. Understanding the Problem Consider a situation where you have a DataFrame that contains pairs of salary ranges as tuples. The goal is to calculate the average salary from these pairs by adding the two numbers and dividing their sum by two. Here’s a quick look at the data structure we are working with: [[See Video to Reveal this Text or Code Snippet]] Each entry in the Salary column represents a tuple with two salary figures. Your task is to compute the average salary from these tuples in the simplest way possible. The Best Approach While iterating over the DataFrame and performing calculations using loops might seem like the initial go-to solution, Pandas offers a more elegant and efficient method. Here’s how you can achieve this in just a few lines of code using the apply method along with a lambda function. Step-by-Step Breakdown Import Libraries: Ensure you have imported Pandas and Numpy at the beginning of your script. [[See Video to Reveal this Text or Code Snippet]] Create Your DataFrame: Set up your DataFrame using the data provided: [[See Video to Reveal this Text or Code Snippet]] Use apply with lambda: Instead of a loop, you can leverage the apply method to compute the mean of each tuple directly. [[See Video to Reveal this Text or Code Snippet]] Check the Output: After running the above code, here’s what your DataFrame should look like: [[See Video to Reveal this Text or Code Snippet]] The output will display the average salary for each salary range: [[See Video to Reveal this Text or Code Snippet]] Benefits of This Approach Readability: Using apply with lambda provides a clear and concise way to perform your desired computation without the clutter of loops. Performance: Pandas is optimized for operations on its data structures, making it more efficient than native Python loops, especially with larger datasets. Code Simplicity: This approach produces less code, reducing the chance for errors and improving maintainability. Conclusion In summary, when faced with the need to add two numbers in a Pandas DataFrame, remember that elegant solutions often exist just a function away. Utilizing apply and lambda allows you to run calculations smoothly, creating a DataFrame that is not just informative but also easy to manage and comprehend. Next time you work with numerical data in Pandas, keep these methods in mind to enhance your coding efficiency! Happy coding!