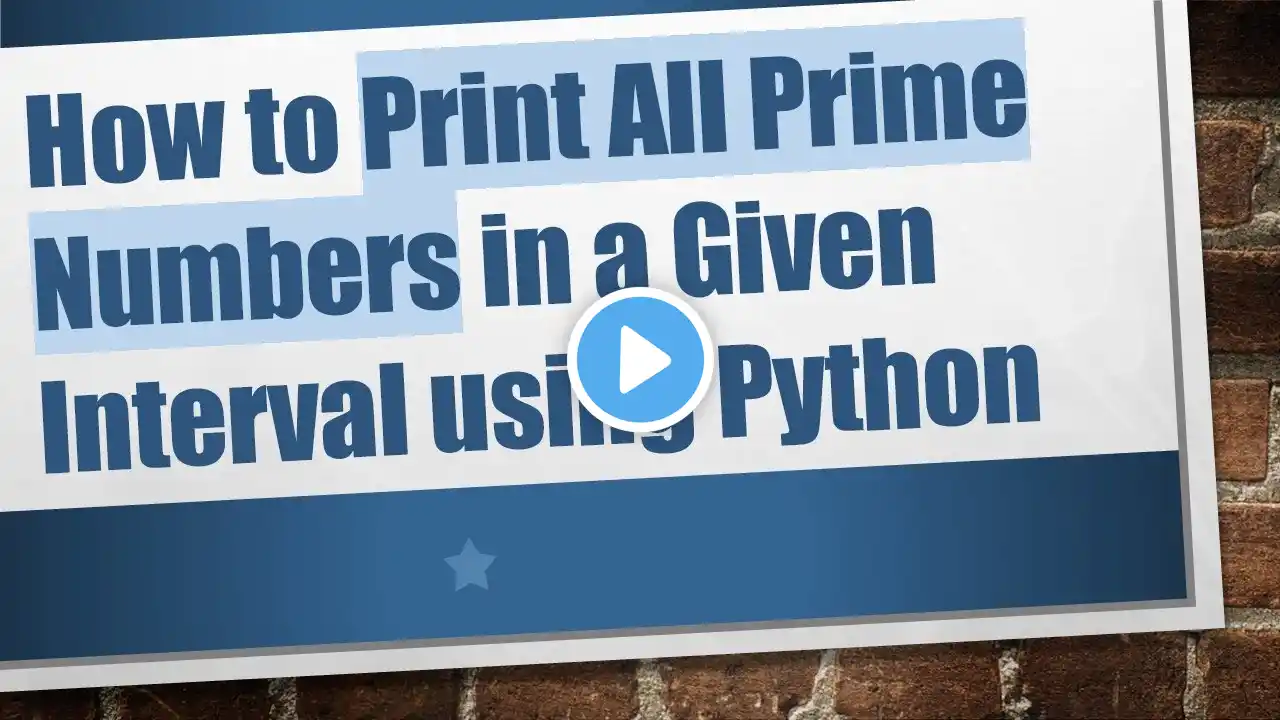
How to Print All Prime Numbers in a Given Interval using Python
Discover how to write a Python program that efficiently identifies and prints all prime numbers within a specified range. Follow our step-by-step guide for better understanding. --- This video is based on the question https://stackoverflow.com/q/74936604/ asked by the user 'Parvathi Pradeep' ( https://stackoverflow.com/u/20580187/ ) and on the answer https://stackoverflow.com/a/74936684/ provided by the user 'Yash Mehta' ( https://stackoverflow.com/u/20172954/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Write a Python program to print all prime numbers in an interval? Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- How to Print All Prime Numbers in a Given Interval using Python Prime numbers are fascinating numbers that have intrigued mathematicians for centuries. They are defined as numbers greater than 1 that have no divisors other than themselves and 1. In this guide, we will tackle the problem of how to print all prime numbers in a specified range (from start to end) using Python. The Problem You want to create a Python program that does the following: Accepts two integers, a start value and an end value Identifies the prime numbers between this range Prints these prime numbers The challenge comes with accurately defining what a prime number is and how to efficiently search for these prime numbers within the specified interval. The Solution Let's break down the solution into manageable parts. Step 1: Define a Function to Check for Prime Numbers First, we need a function that will determine if a number is prime. We can achieve this with a straightforward approach: First, any number less than or equal to 1 should not be considered a prime number. For numbers greater than 1, check that the number cannot be divided evenly by any number from 2 to one less than itself. Here’s an improved version of the is_prime function: [[See Video to Reveal this Text or Code Snippet]] Step 2: Create a Function to Print Prime Numbers Next, we define another function that generates the list of prime numbers in the specified range. This function: Iterates from start to end Calls the is_prime function to check each number Collects all prime numbers in a list Returns the list of prime numbers Here's how that looks in code: [[See Video to Reveal this Text or Code Snippet]] Step 3: Gather User Input and Execute the Functions To make our program interactive, we will take input from the user: [[See Video to Reveal this Text or Code Snippet]] Handling Known Bugs Be sure to include proper closure in input statements so they'll execute correctly. We only needed to check numbers up to n-1 for primality. For better readability, we stored the results in a variable before printing. Example Usage When you run the program and input: [[See Video to Reveal this Text or Code Snippet]] You should see the output: [[See Video to Reveal this Text or Code Snippet]] Final Note The print_primes function works inclusively for the start value but exclusively for the end value. If you want the end value to be included, simply change the range to go up to end + 1 in the for loop. Conclusion With these steps, you now have a fully functional Python program that prints all prime numbers within a specified interval! This exercise not only helps in understanding prime numbers, but it also enhances your basic programming skills in Python. Feel free to experiment with the code, tweak the functions, and learn more about how numbers work. Happy coding!