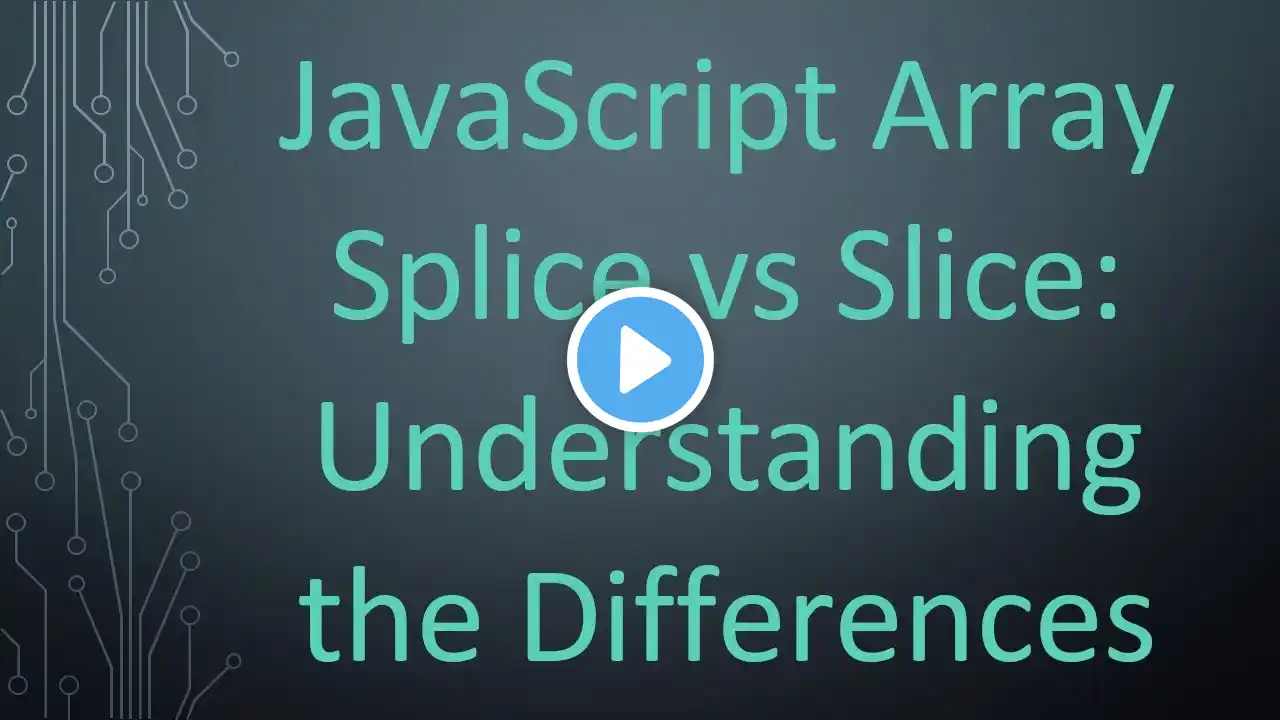
JavaScript Array Splice vs Slice: Understanding the Differences
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you. --- Summary: Discover the key differences between JavaScript array methods `splice` and `slice`, their syntax, use cases, and best practices for effective coding. --- JavaScript Array Splice vs Slice: Understanding the Differences JavaScript provides various array methods to manipulate and manage data efficiently. Two commonly used methods for managing arrays are splice and slice. While they may seem similar at first glance, they serve distinctly different purposes. This guide will explore their functionalities, syntax, and when to use each method. Splice Method The splice method modifies the original array by removing or replacing existing elements and/or adding new elements. Here's the syntax for the splice method: [[See Video to Reveal this Text or Code Snippet]] Parameters: start: Index at which to start changing the array. deleteCount: Number of elements to remove from the array. item1, item2, ..., itemN (optional): New elements to add to the array starting from the start index. Example: [[See Video to Reveal this Text or Code Snippet]] In this example, starting at index 1, it removes 2 elements ("banana" and "cherry") and adds 2 new elements ("blueberry" and "cantaloupe"). Slice Method The slice method returns a shallow copy of a portion of an array into a new array object. It does not modify the original array. Here's the syntax for the slice method: [[See Video to Reveal this Text or Code Snippet]] Parameters: begin: Index at which to begin extraction. The extraction starts from this index. end (optional): Index at which to end extraction. The element at this index is not included in the returned array. Example: [[See Video to Reveal this Text or Code Snippet]] In this example, the slice method starts the extraction at index 1 and ends at index 3. It includes elements at index 1 and 2 but not at index 3. Key Differences Modification vs Non-Modification: The splice method modifies the original array, whereas the slice method returns a new array and does not alter the original array. Insertion: The splice method can add new elements to the array; slice cannot. Return Value: The splice method returns an array of the deleted elements, while the slice method returns a portion of the original array as a new array. Use Cases Use splice when you need to remove specific items from an array and potentially add new ones, while also modifying the original array. Use slice when you need to create a copy of a portion of an array without altering the original array. Conclusion Understanding the differences between splice and slice is crucial for effective array manipulation in JavaScript. Both methods serve unique purposes and knowing when to use each can help you manage array data more efficiently in your applications. Happy coding!