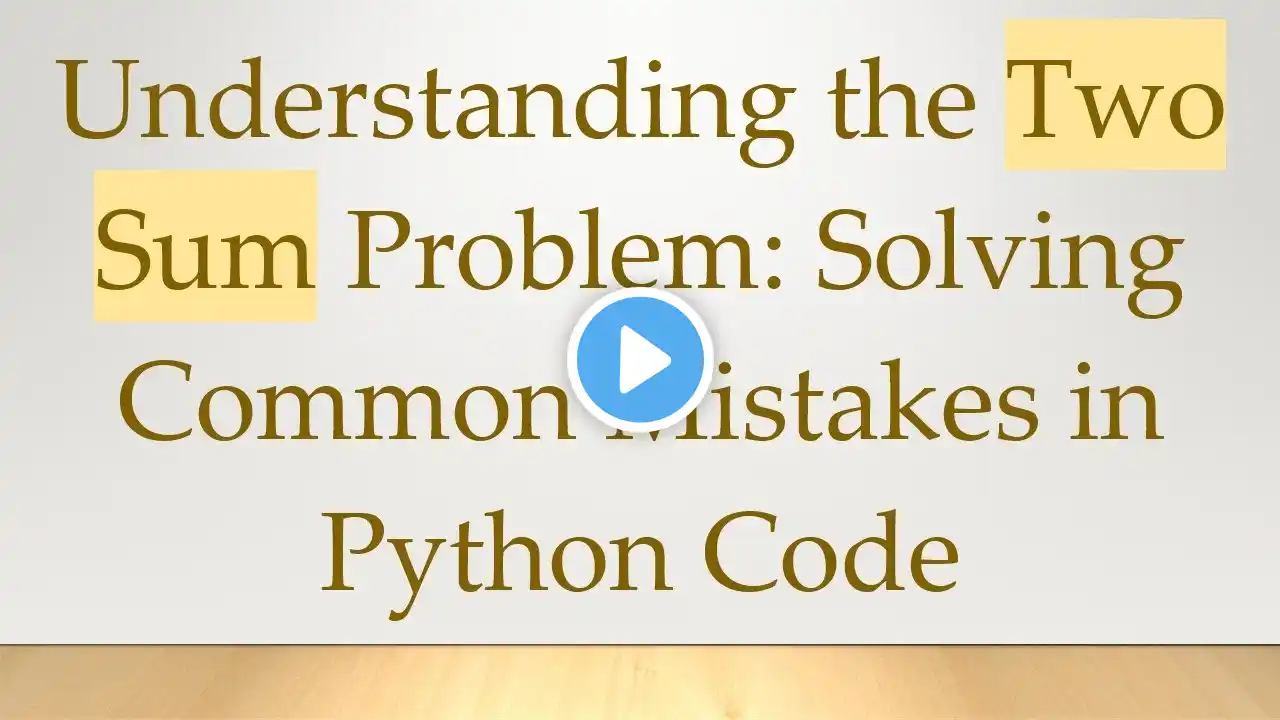
Understanding the Two Sum Problem: Solving Common Mistakes in Python Code
Learn why your solution to the `Two Sum` problem might fail, specifically with input [3, 2, 3]. Discover the best practices to fix your Python code efficiently. --- This video is based on the question https://stackoverflow.com/q/69136892/ asked by the user 'wehguidg' ( https://stackoverflow.com/u/15918635/ ) and on the answer https://stackoverflow.com/a/69137007/ provided by the user 'Barmar' ( https://stackoverflow.com/u/1491895/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Why is my answer to the Two Sum problem failing with [3,2,3]? Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- Solving the Two Sum Problem: A Common Pitfall If you've ever worked on coding challenges, you've probably encountered the Two Sum problem: finding two numbers in an array that add up to a specific target. It's a classic question that tests your understanding of arrays and indexing, but even seasoned developers can run into issues. One common source of confusion arises when handling duplicate numbers in arrays, as many have discovered with the input [3, 2, 3]. In this guide, we will break down the issues surrounding this input, analyze the initial code, and explore the correct approach to solve the problem effectively. The Problem Statement Given an array of integers, and a target integer, the goal is to find the indices of the two numbers that add up to the target. For the input [3, 2, 3], if the target is 9, the expected output should be the indices of the two 3s, which are 0 and 2. However, if your implementation fails with this input, let’s examine why this could happen. The Code Breakdown Here’s the code that the user had initially implemented: [[See Video to Reveal this Text or Code Snippet]] Issues with the Original Code Using nums.index(): The index() method returns the index of the first occurrence of a number in the list. This means when you call nums.index(i), it will always return the index of the first 3, even if you're looking for a different 3 in the list. Inefficient Nested Loops: The code uses three nested loops, which not only makes it inefficient but also leads to complexity in finding elements. Count and List Management: The code keeps track of a count variable and an accumulating list, which is unnecessary since you only need to return the first valid index pair. A More Efficient Solution The key to a more elegant and effective solution is to avoid redundant loops and to properly utilize indexing. Here's a refined version of the Two Sum implementation that addresses all the above issues: [[See Video to Reveal this Text or Code Snippet]] Explanation of the New Code Using enumerate: This function is now used within both loops, allowing you to manage indices effectively. By starting the inner loop with i + 1, we ensure that we only compare the current element with those that come after it. This automatically handles the issue of double counting or using the same element twice. Simplified Return Statement: The function returns the indices as soon as it finds a valid pair, eliminating the need for a separate counter or list management. Readability and Efficiency: The updated solution is not only cleaner but also runs in O(n^2) time complexity, which is acceptable for a relatively small list such as [3, 2, 3]. Conclusion The Two Sum problem serves as a great reminder that simple mistakes in indexing can lead to incorrect results. By revising our approach and utilizing Python's capabilities efficiently, we can solve these challenges effectively. So next time you tackle similar problems, remember to take care with how you manage your indices and loops, and your code will be robust and reliable. Happy coding, and remember that it’s okay to make mistakes—they’re often the best learning experiences!