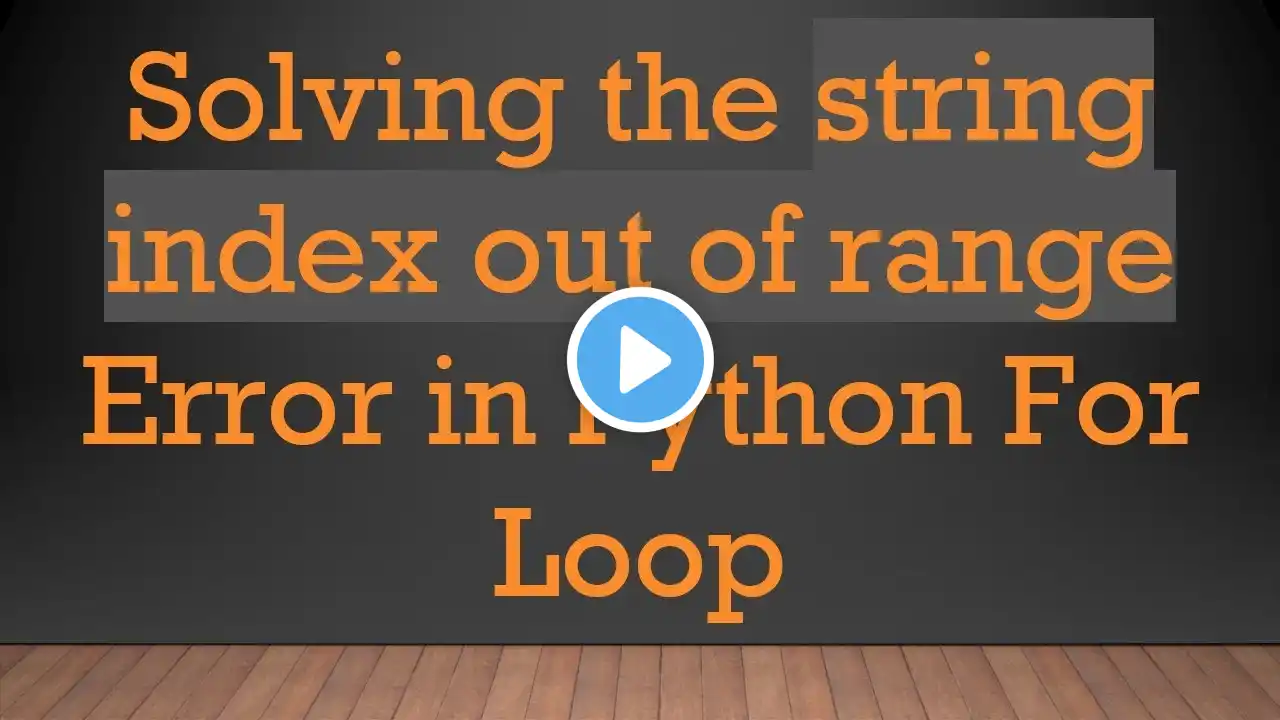
Solving the string index out of range Error in Python For Loop
A guide on fixing the `string index out of range` error in Python when finding the longest common prefix among strings in a list. Get step-by-step instructions to refine your function and avoid this common issue! --- This video is based on the question https://stackoverflow.com/q/76622067/ asked by the user 'Simply Code' ( https://stackoverflow.com/u/14818305/ ) and on the answer https://stackoverflow.com/a/76622162/ provided by the user 'Freeman' ( https://stackoverflow.com/u/252518/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: string index out of range error at end of for loop Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- Solving the string index out of range Error in Python For Loop As a Python programmer, encountering errors is part of the journey. One such common error you might face is the dreaded string index out of range message. If you've stumbled upon this while working on a function to find the longest common prefix among strings in a list, you're not alone! In this guide, we'll discuss this error and walk through a refined solution, ensuring that you have a strong grasp of how to tackle such issues in your Python code. Understanding the Issue Consider the following function designed to find the longest common prefix from a list of strings: [[See Video to Reveal this Text or Code Snippet]] While attempting to run this function, you might encounter an error like this: [[See Video to Reveal this Text or Code Snippet]] This typically happens because the inner loop tries to access an index in the string that doesn't exist, especially when the length of the current string i or the long_comm has changed or is shorter than expected. Breakdown of the Error When the loop runs, it compares the characters of each string one by one. If it encounters a situation where one string is shorter than long_comm, it mistakenly tries to access an index that goes beyond the shorter string's length, leading to an IndexError. The Solution To fix this issue, a better approach is needed. Here's a revised version of the function that addresses the error systematically: [[See Video to Reveal this Text or Code Snippet]] Explanation of the Revised Code Initialize: The function starts with the first string in the list as the potential longest common prefix. Iterate: Loop through each string i in the input list strs. Updated Variable: Create a new variable updated_long_comm that will collect characters that match the current prefix. Character Comparison: The inner loop iterates character by character only up to the length of the shorter prefix. If a mismatch is found (i[j] != long_comm[j]), the loop breaks, ensuring you do not exceed string bounds. Prefix Assignment: After checking all characters for the current string, assign updated_long_comm to long_comm. Result This refined function effectively prevents the string index out of range error by checking string lengths before performing comparisons and thus allows for accurate determination of the longest common prefix across the strings. Conclusion The string index out of range error can be a hurdle in your coding journey, but with a better understanding of string manipulation and control flow in Python, you can overcome it. By implementing the above solution and keeping these principles in mind, you're on your way to writing more robust Python code. Good luck with your programming!