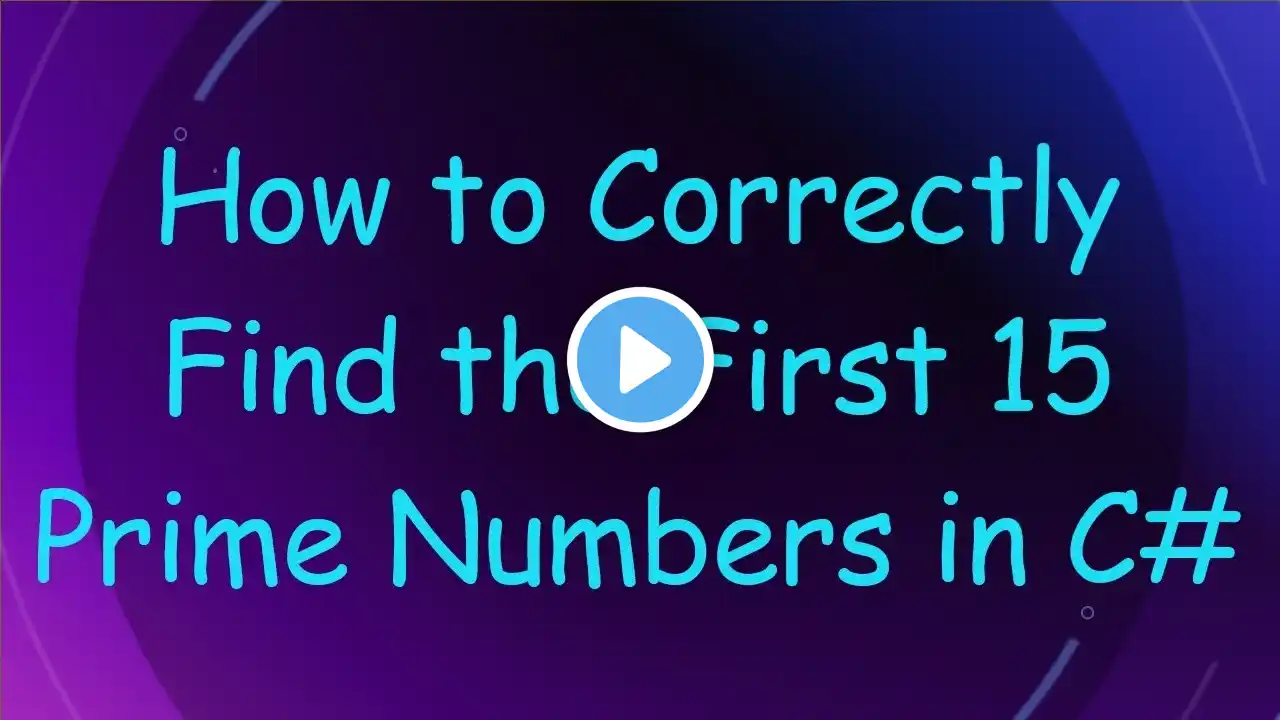
How to Correctly Find the First 15 Prime Numbers in C#
Discover the common mistake in creating an array of the first 15 prime numbers in C-. Improve your logic and optimize your code with our detailed guide! --- This video is based on the question https://stackoverflow.com/q/73926096/ asked by the user 'philiptarum' ( https://stackoverflow.com/u/20127110/ ) and on the answer https://stackoverflow.com/a/73926225/ provided by the user 'Karel Křesťan' ( https://stackoverflow.com/u/9472426/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: c- array in prime numbers - Where is my mistake? Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- How to Correctly Find the First 15 Prime Numbers in C- In programming, especially when dealing with algorithms and numerical calculations, it's common to run into issues with logic and function behaviors. One such scenario occurs when trying to identify prime numbers. If you're coding in C- and attempting to generate an array filled with the first 15 prime numbers, you might encounter a misstep. This guide explores a common mistake and provides strategies to optimize your solution. Understanding the Problem The goal here is simple: create an array that contains the first 15 prime numbers. However, as you dive into your C- code, everything may not work as expected. Let's take a closer look at the flawed implementation and identify the errors. The Original Code Here’s a brief recap of the provided code: [[See Video to Reveal this Text or Code Snippet]] The Prime Check Function Additionally, there’s a method to check if a number is prime: [[See Video to Reveal this Text or Code Snippet]] Identifying the Mistake Upon examining the code, the primary issue resides within the IsPrime function. Recognizing the Logic Flaw The code is designed to return true if the number is not prime (because it returns true on finding a divisor). The correct logic should return false when a number is divisible (indicating it is not prime) and true otherwise. Required Modification Here’s how you should modify the IsPrime function: [[See Video to Reveal this Text or Code Snippet]] Optimization Suggestions Now that the fundamental error is addressed, let’s further enhance the code for improved performance. Optimizing the Prime Check Limit the Loop: Instead of checking divisibility up to number - 1, you can limit it to the square root of the number. This reduces the number of iterations significantly. Skip Even Numbers: After checking for the number 2, you can skip even numbers altogether in the main loop, as they cannot be prime (except for 2). Refining the Main Loop Rather than incrementing i by 1, increment it by 2 after checking for 2. This logic will enhance performance, especially for larger ranges. [[See Video to Reveal this Text or Code Snippet]] Conclusion Identifying and correcting logical errors is a vital part of programming. By addressing the mistake in the IsPrime function and optimizing both it and the overall structure of your code, you can efficiently fill your array with the first 15 prime numbers. Building such algorithms can be challenging but also highly rewarding. Keep experimenting with optimizations, and you'll find that your coding confidence will grow with each step. Remember, effective coding involves not just solving problems, but also continuously refining and enhancing the solutions you arrive at.