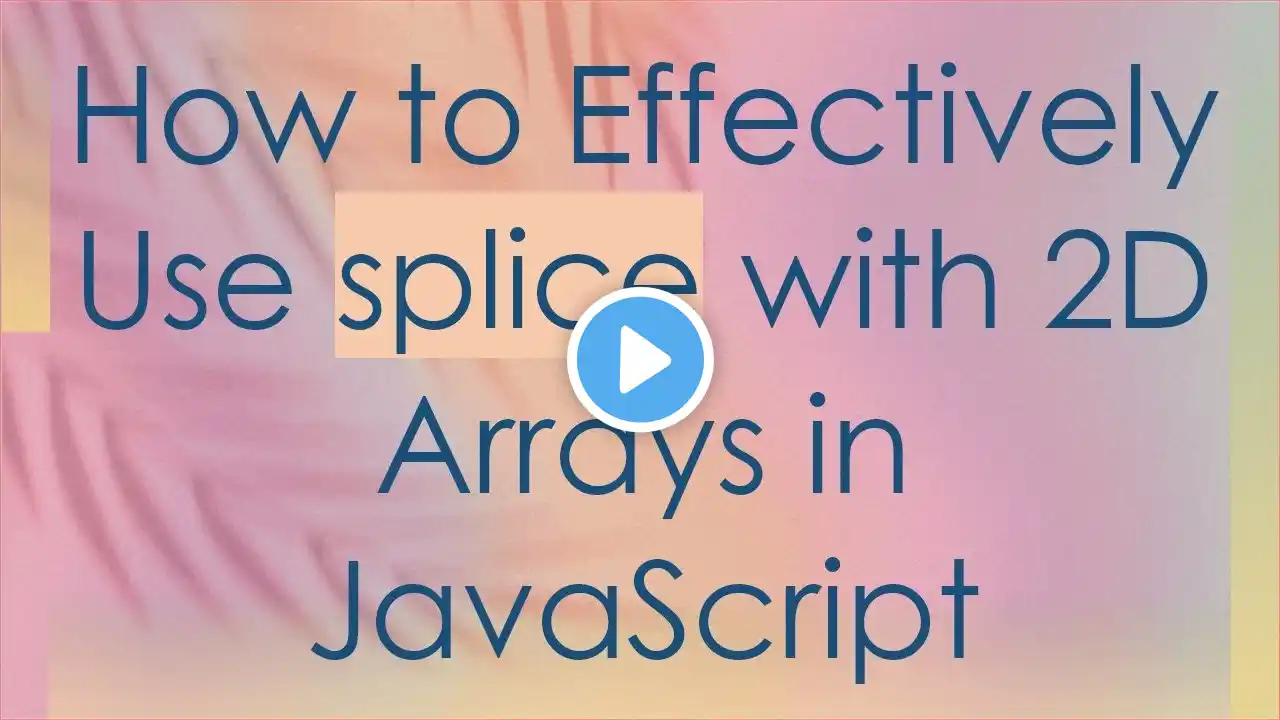
How to Effectively Use splice with 2D Arrays in JavaScript
Learn how to delete specific rows from a 2D array in JavaScript using the `splice` method, and understand why iterating backward is key to success. --- This video is based on the question https://stackoverflow.com/q/72853886/ asked by the user 'Yuval Shamir' ( https://stackoverflow.com/u/19349995/ ) and on the answer https://stackoverflow.com/a/72854937/ provided by the user 'Robin Mackenzie' ( https://stackoverflow.com/u/765395/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: using splice inside 2D Array in Javascript Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- Mastering the Use of splice in 2D Arrays with JavaScript When working with arrays in JavaScript, especially 2D arrays, you might encounter situations where you need to remove certain elements – like entire rows in a 2D array. A common use case is to delete rows that contain a specific number of a certain value, such as the number of 1s in a row. In this guide, we will explore a scenario where you need to delete rows with 5 or more 1s, and we will present a solution that will help you do this efficiently while avoiding common pitfalls. The Problem You have a 2D array (an array of arrays) and you're trying to remove any rows that contain 5 or more occurrences of the number 1. You attempted to use the splice method, but encountered an issue with the way the loop was structured, leading to unintended behavior when the array is modified during iteration. This kind of modification while iterating can lead to skipped rows and unexpected results because the indices change as rows are removed. Let's take a closer look at the original code snippet provided: [[See Video to Reveal this Text or Code Snippet]] The Solution: Iterate Backwards The key to successfully using splice in this situation is to iterate backwards through the array. By starting from the end and working your way to the front, you prevent any index shifting from affecting rows that have not yet been evaluated. Below, we’ll modify the code accordingly: Updated Code Example [[See Video to Reveal this Text or Code Snippet]] Code Breakdown Initialization: The 2D array a is initialized to have row counts of 7 and column counts of 10, filled with 0s initially. Populating the Array: Specific entries are set to 1 to create rows with different counts of 1s. Iterating Backwards: The loop starts from the last index (a.length - 1) and decrements until it reaches 0. This prevents index issues when removing a row. Counting 1s: For each row, we count how many times 1 appears. Conditionally Removing Rows: If a row has more than 4 ones, we remove it with splice(j, 1). Output: Finally, we log both the original and filtered arrays for verification. Conclusion Using the splice method in a 2D array can be tricky, especially when modifying the array while looping through it. By iterating backwards, you can effectively manage the array indices and remove the unwanted rows without skipping any rows. This solution should help you efficiently filter your 2D arrays in JavaScript! Happy coding!