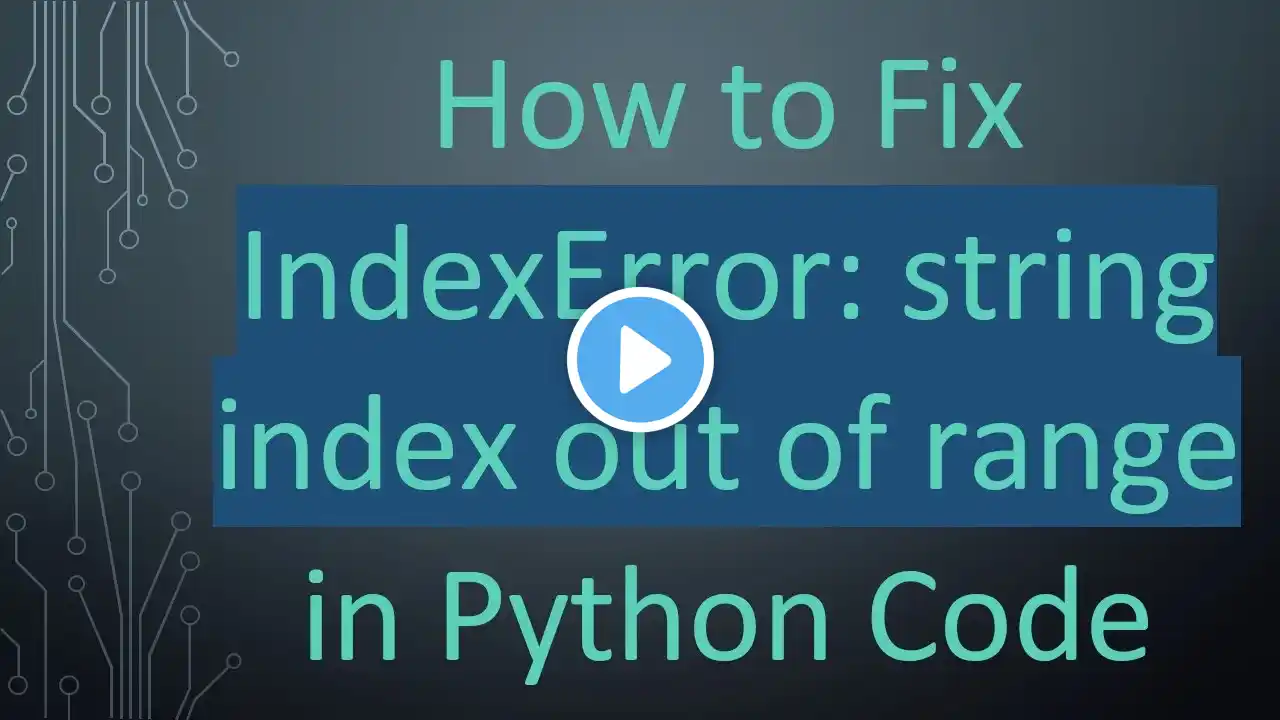
How to Fix IndexError: string index out of range in Python Code
Learn how to troubleshoot and fix the common `IndexError: string index out of range` error in your Python programs. This guide provides a step-by-step breakdown of the issue and offers practical solutions. --- This video is based on the question https://stackoverflow.com/q/78169557/ asked by the user 'sokunn S' ( https://stackoverflow.com/u/23628633/ ) and on the answer https://stackoverflow.com/a/78169796/ provided by the user 'independent_bit1256' ( https://stackoverflow.com/u/23625599/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, comments, revision history etc. For example, the original title of the Question was: Why does it show IndexError: string index out of range? Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- Understanding and Fixing the IndexError: string index out of range in Python When working with strings in Python, it's common for beginners to encounter the IndexError: string index out of range. This error can be frustrating, especially when you're trying to achieve a specific functionality in your code. In this post, we'll explore a particular case that illustrates this error and provide a clear solution to help you avoid it in your own coding endeavors. The Problem Imagine you have a simple function that compares numbers in a string and calculates a score based on their relationships. For example, if your input string is 5823, the function is designed to score the sequence based on whether a number is smaller than the one that follows it. Here's the initial code you might have: [[See Video to Reveal this Text or Code Snippet]] However, upon running this code, you might encounter an IndexError. Why does this happen? Explanation of the Error The crux of the problem lies in the variable maxs, which is set to length + 1. This is where the error originates: The variable length is derived from the length of the input string. When you declare maxs, it inadvertently allows the index n to exceed the valid range of the string. In Python, string indices start at 0 and go up to n-1 for a string of length n. Therefore, if you attempt to access list[n] when n equals length, you'll step outside the bounds of the string and trigger an IndexError. Example Breakdown Input: 5823 → The length is 4. Setting maxs: The variable maxs equals length + 1 → 5. Iteration: This allows the loop to potentially run for 5 iterations, meaning n could reach 4, which is invalid. Solution Steps 1. Eliminate the maxs Variable The simplest fix is to remove the maxs variable altogether and update your loop condition. Use the length directly in your loop. 2. Update the Loop Condition Change your loop to iterate based on the actual length of the list. You can set it to run while n < length - 1: [[See Video to Reveal this Text or Code Snippet]] 3. Simplify Redundant Variables In your code, the variable test is unnecessary as you can achieve the same functionality using just n. Here’s the Revised Code Here’s a cleaned-up version of your function using the changes mentioned: [[See Video to Reveal this Text or Code Snippet]] Conclusion By following these steps, you can effectively avoid the IndexError: string index out of range in your Python programs. Always remember that string indices start at 0 and be mindful of iterating beyond their limits. If you run into similar issues in the future, you now have the tools to diagnose and fix the problem quickly. Happy coding!