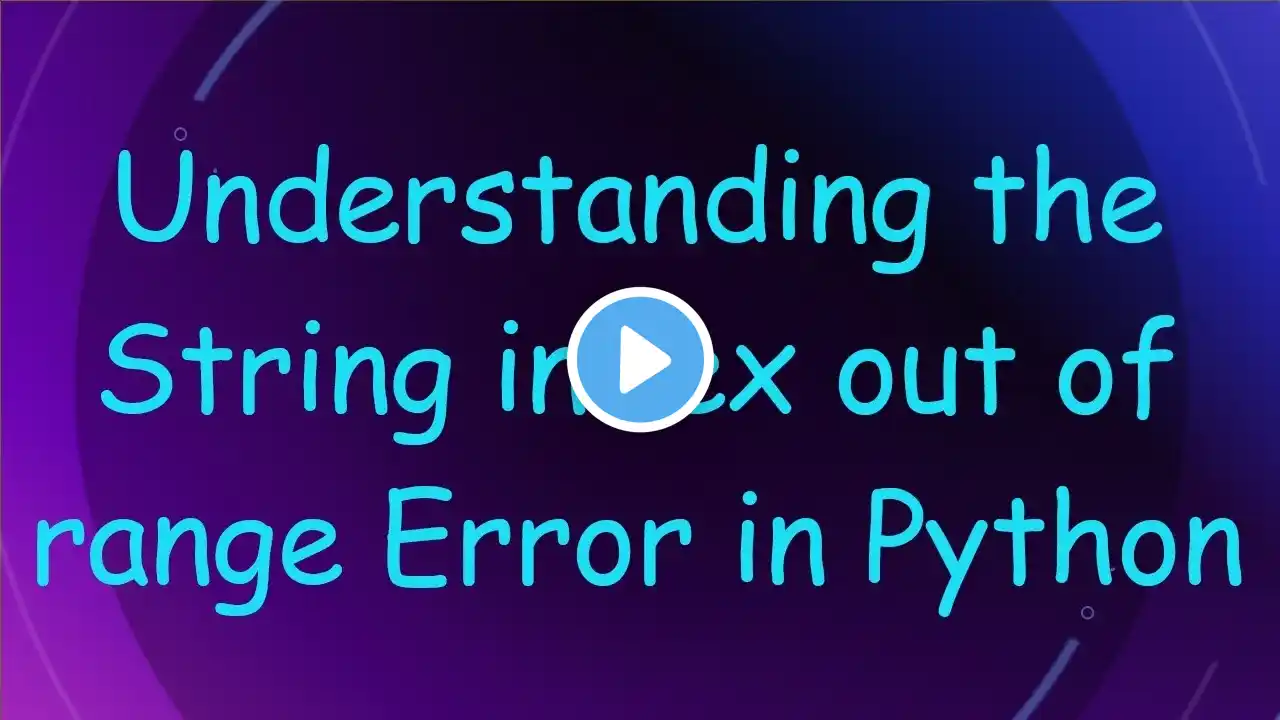
Understanding the String index out of range Error in Python
Discover how to fix the 'String index out of range' error in Python when dropping vowels from a phrase with our step-by-step solution. --- This video is based on the question https://stackoverflow.com/q/73393399/ asked by the user 'RKSiegel' ( https://stackoverflow.com/u/2121886/ ) and on the answer https://stackoverflow.com/a/73393556/ provided by the user 'Arifa Chan' ( https://stackoverflow.com/u/19574157/ ) at 'Stack Overflow' website. Thanks to these great users and Stackexchange community for their contributions. Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: 'String index out of range' error and I don't understand why Also, Content (except music) licensed under CC BY-SA https://meta.stackexchange.com/help/l... The original Question post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license, and the original Answer post is licensed under the 'CC BY-SA 4.0' ( https://creativecommons.org/licenses/... ) license. If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com. --- Understanding the String index out of range Error in Python: A Complete Guide When working with strings in Python, you might encounter a frustrating error known as String index out of range. If you've ever tried to manipulate a string and run into this issue, you're not alone! In this guide, we'll tackle this problem head-on, providing you not only with the solution but also with the necessary context to help you grasp why this error occurs in the first place. The Problem Imagine you’re trying to create a simple program that takes a user's input, removes all vowels from that input, and then prints the new phrase. Sounds easy, right? However, if you use code similar to the snippet below, you might receive an error that leaves you scratching your head: [[See Video to Reveal this Text or Code Snippet]] The Error The specific error you might encounter is: [[See Video to Reveal this Text or Code Snippet]] This typically means that when your code tries to access an index in a string, that index doesn't exist. At first glance, it seems like you've limited the index to the length of the phrase with range(len(phrase)). So, what’s causing the error? Let's break it down. Understanding the Cause In your code, you are modifying the phrase string inside the loop. When you replace a character in phrase, its length changes. This means that when the loop tries to access further indices, they may no longer exist, leading to the index out of range error. This is where your problem stems from. The Solution Fortunately, there’s a simpler and more effective way to accomplish the task of removing vowels from a string. Instead of looping through indices, we can loop directly through the characters in the string. Here's how you can rewrite your code effectively: [[See Video to Reveal this Text or Code Snippet]] Key Changes Made: Loop Through Characters: Replace for i in range(len(phrase)): with for i in phrase:. This way, you are directly accessing the characters without needing to worry about index values. Simplified Replacement: By maintaining the integrity of your original string, you eliminate the risk of causing an index error when replacing characters. Additional Tips Use List Comprehension: For an even cleaner approach, consider using list comprehension to build a new phrase without vowels: [[See Video to Reveal this Text or Code Snippet]] Regex for More Complexity: For more complex string manipulations, consider using the re module, which provides powerful string searching and manipulation capabilities using regular expressions. Conclusion In conclusion, the String index out of range error in Python often occurs when you're attempting to access an index that no longer exists due to modifications in the string length. By understanding this principle and exploring more straightforward ways to manipulate strings—like removing vowels using character loops—you can avoid these pitfalls. Happy coding!